When writing Bash scripts, it is often necessary to pass data to the script during execution without modifying the script itself. This is where command-line arguments come into play. By utilizing these arguments, you can create flexible and dynamic scripts that operate differently based on user input.
Understanding Command Line Arguments
Command-line arguments are parameters that are passed to a script when it is executed. In Bash, these arguments are represented by special variables:
$0
– The name of the script itself.$1, $2, $3, ...
– Positional parameters representing arguments from the command line.$#
– The total number of arguments passed.$@
– All arguments as a single string.$*
– Similar to$@
, but with differences in behavior when quoted.$?
– The exit status of the last command executed.
How to Use Command Line Arguments in a Bash Script
To illustrate how arguments work in a Bash script, let’s look at a simple example. Suppose we have the following script:
#!/bin/bash
echo "Script name: $0"
echo "First argument: $1"
echo "Second argument: $2"
echo "Total number of arguments: $#"
Save this script as example.sh
and make it executable using:
chmod +x example.sh
Now, execute the script with some arguments:
./example.sh Hello World
The output will be:
Script name: ./example.sh
First argument: Hello
Second argument: World
Total number of arguments: 2
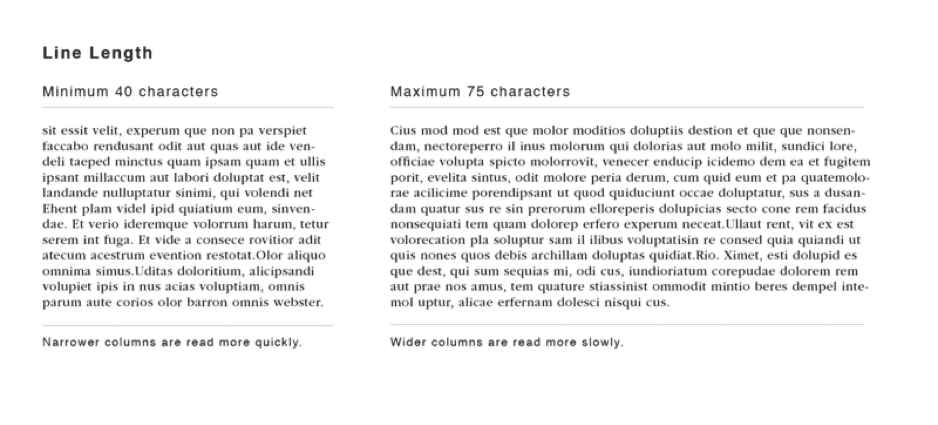
Iterating Over Multiple Arguments
If your script needs to process multiple arguments dynamically, a loop is useful. Here’s how you can iterate over all arguments:
#!/bin/bash
echo "All arguments passed:"
for arg in "$@"; do
echo "$arg"
done
Using Named Arguments with Flags
For more structured scripts, you may want to provide named arguments using flags. The getopts
command helps with this:
#!/bin/bash
while getopts "n:a:" opt; do
case "$opt" in
n) name=$OPTARG ;;
a) age=$OPTARG ;;
?) echo "Usage: $0 -n [name] -a [age]" ;;
esac
done
echo "Name: $name"
echo "Age: $age"
Running this script as follows:
./script.sh -n Alice -a 30
Produces:
Name: Alice
Age: 30
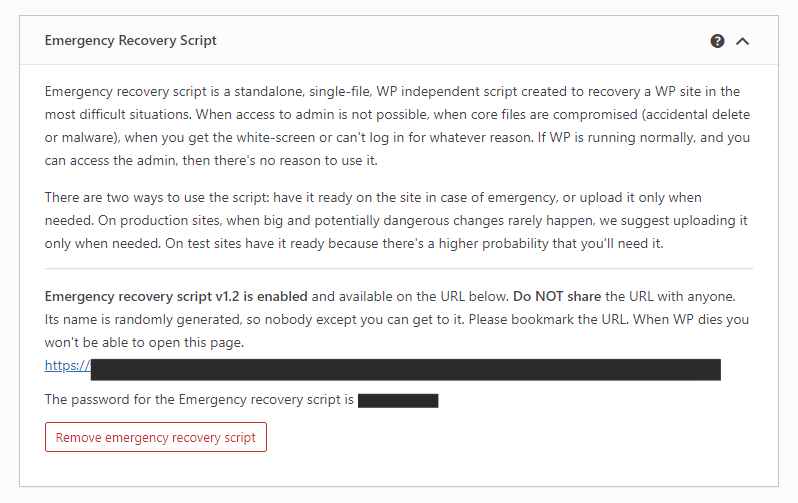
Handling Missing Arguments
It is important to check whether required arguments are provided. Here’s an example of handling missing arguments gracefully:
#!/bin/bash
if [ $# -lt 2 ]; then
echo "Usage: $0 "
exit 1
fi
echo "Argument 1: $1"
echo "Argument 2: $2"
If the user provides fewer than two arguments, the script displays an error message and exits with a non-zero status.
Using Shift to Process Arguments
The shift
command can be used to discard processed arguments and move to the next one:
#!/bin/bash
while [ $# -gt 0 ]; do
echo "Processing argument: $1"
shift
done
This script will process each argument sequentially and remove it from the list as it moves forward.
Conclusion
Incorporating command-line arguments allows Bash scripts to be more interactive and versatile. By using positional parameters, loops, and argument flags, you can create scripts that handle a variety of inputs effectively. Learning these techniques is essential for writing powerful automation scripts in any Linux environment.