Passing a file argument to a Bash script is an essential skill for any Linux user who wants to create flexible and efficient scripts. By allowing scripts to accept file inputs dynamically, you can process different files without modifying the script each time. In this article, we will explore how to pass a file argument to a Bash script using a terminal command and demonstrate best practices for handling file input.
Understanding Command-Line Arguments in Bash
Bash scripts can accept arguments from the command line when they are executed. These arguments are accessible within the script using special variables:
$0
– The name of the script itself$1
,$2
, etc. – The positional parameters representing arguments passed to the script$#
– The number of arguments passed$@
– All arguments as a list
When passing a file as an argument, it will be available as $1
if it is the first argument.
Creating a Bash Script to Accept a File
Let us create a simple Bash script that takes a file as an argument and displays its contents.
#!/bin/bash # Check if an argument was provided if [ $# -eq 0 ]; then echo "Usage: $0 filename" exit 1 fi # Assign the first argument to a variable file=$1 # Check if the file exists if [ ! -f "$file" ]; then echo "Error: File '$file' does not exist." exit 1 fi # Display the content of the file cat "$file"
Save this script as readfile.sh
and give it execution permission using:
chmod +x readfile.sh
Passing a File Argument from the Terminal
To pass a file to this script, use the following command:
./readfile.sh myfile.txt
Replace myfile.txt with the actual file you want to pass. The script will check if the file exists before displaying its contents.
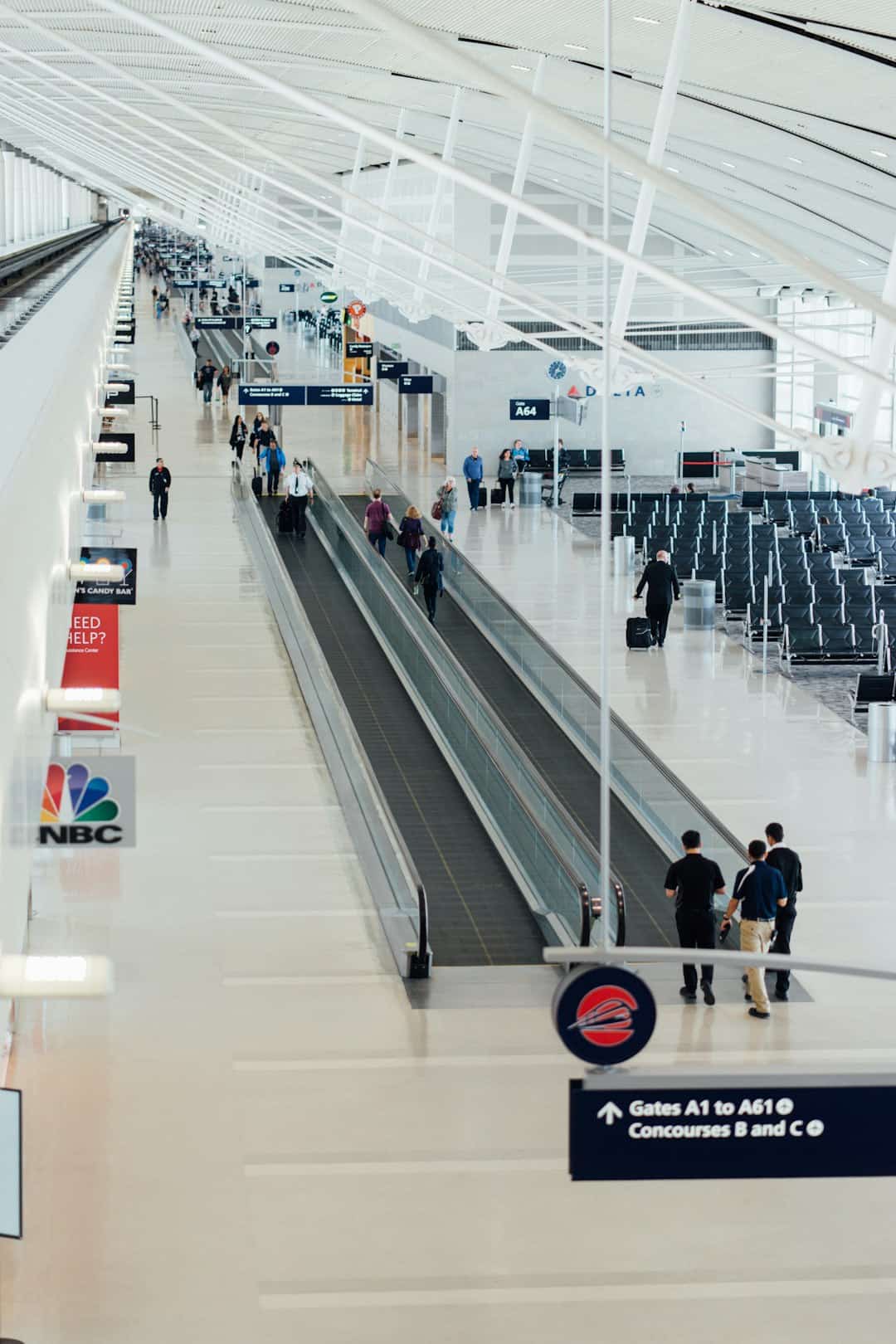
Handling Multiple File Arguments
If you need to process multiple files, you can modify the script to loop through all provided arguments:
#!/bin/bash # Check if at least one argument is provided if [ $# -eq 0 ]; then echo "Usage: $0 file1 [file2 ...]" exit 1 fi # Loop through all file arguments for file in "$@"; do if [ -f "$file" ]; then echo "Displaying contents of: $file" cat "$file" echo "" else echo "Error: File '$file' does not exist." fi done
Now, you can pass multiple files:
./readfile.sh file1.txt file2.txt file3.txt
Best Practices for Handling File Arguments
When writing Bash scripts that accept file arguments, follow these best practices:
- Validate inputs: Always check if the file exists and is accessible before processing.
- Use quotation marks: Wrap variables in double quotes (
"$file"
) to handle filenames with spaces correctly. - Handle errors gracefully: Provide meaningful error messages and exit codes to help users troubleshoot issues.
- Consider alternate input methods: If your script needs more flexibility, allow input via standard input (
stdin
) as well.
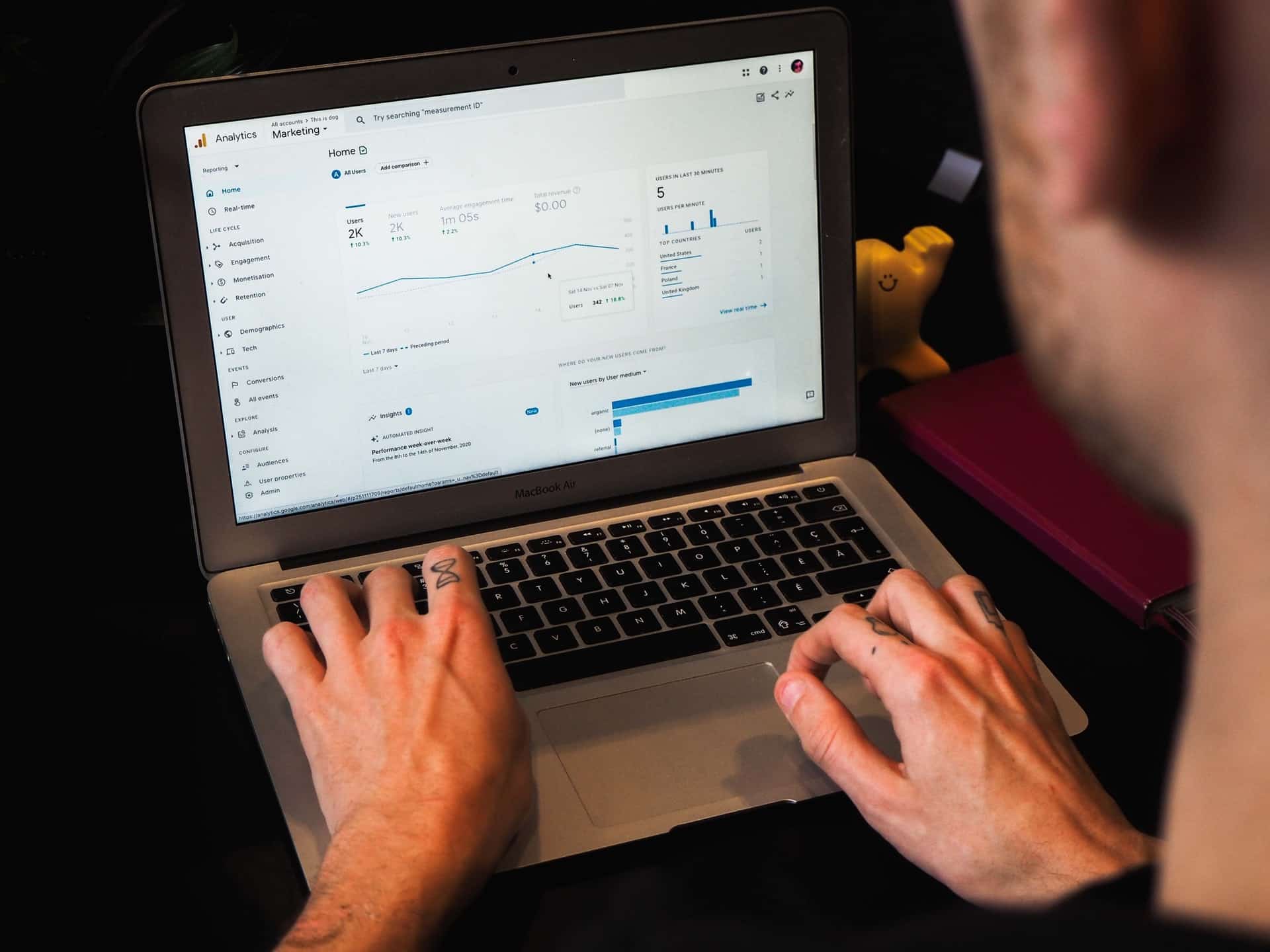
Advanced Techniques: Accepting File Paths with Flags
For more advanced scripts, you may want to use flags instead of positional arguments. Using the getopts
command, your script can accept file arguments more flexibly:
#!/bin/bash while getopts "f:" opt; do case "$opt" in f) file="$OPTARG" ;; *) echo "Usage: $0 -f filename"; exit 1 ;; esac done if [ -z "$file" ]; then echo "Error: No file provided." exit 1 fi if [ ! -f "$file" ]; then echo "Error: File '$file' does not exist." exit 1 fi cat "$file"
Execute the script with:
./readfile.sh -f myfile.txt
Conclusion
Passing a file argument to a Bash script is a straightforward yet powerful feature. Whether using positional parameters or flags, ensuring proper validation and error handling is key. By mastering this technique, you can create flexible scripts that handle file inputs efficiently in Linux. Keep experimenting with different approaches, and you’ll soon become proficient in Bash scripting.