“`html
Typing indicators on Discord add a level of interactivity, letting users know when someone is composing a message. By default, Discord includes a built-in typing indicator in direct messages and group chats, but for servers, this feature is not available natively. However, server administrators can utilize bots and custom scripts to implement typing indicators in text channels.
Why Add Typing Indicators?
Typing indicators can enhance engagement in Discord communities by:
- Giving users visual feedback that someone is about to send a message.
- Improving conversation flow by preventing unintended message overlaps.
- Creating a more dynamic and interactive chat experience.
Methods to Add Typing Indicators on a Discord Server
Several techniques allow the implementation of typing indicators. The most common methods involve using Discord bots and API functions.
1. Using a Discord Bot
Many Discord bots offer additional functionalities, including typing indicators. One such example is using a bot built with Discord.js. To create a basic typing indicator bot, follow these steps:
- Set Up a Discord Bot: Visit the Discord Developer Portal and create a new bot.
- Install Dependencies: If using Node.js, install Discord.js by running:
npm install discord.js
- Write a Simple Typing Indicator Script: Here is an example code snippet:
const { Client, GatewayIntentBits } = require('discord.js'); const client = new Client({ intents: [GatewayIntentBits.Guilds, GatewayIntentBits.GuildMessages] }); client.on('messageCreate', async (message) => { if (!message.author.bot) { await message.channel.sendTyping(); } }); client.login('YOUR_BOT_TOKEN');
This script makes the bot simulate typing every time a new message is sent in the server.
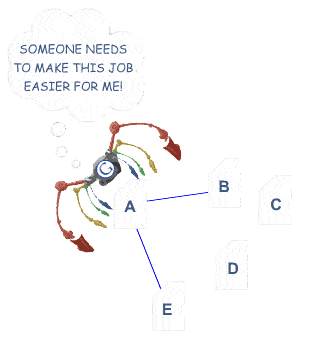
2. Using Discord API’s sendTyping()
Function
For more direct control, developers can integrate the sendTyping()
feature into their custom bot using the Discord API. This method ensures that whenever a user interacts with the bot, it will display a typing indicator before responding.
3. Using Third-Party Bots
If coding is not an option, there are pre-existing bots that provide typing indicators. Some popular choices include:
- MEE6: A popular bot with customization options.
- Dyno Bot: Includes moderation and typing indicator features.
- Nadeko: A multi-purpose bot offering typing-related functions.
To use these, invite the bot to your server, configure settings through the dashboard, and enable typing indicators where available.
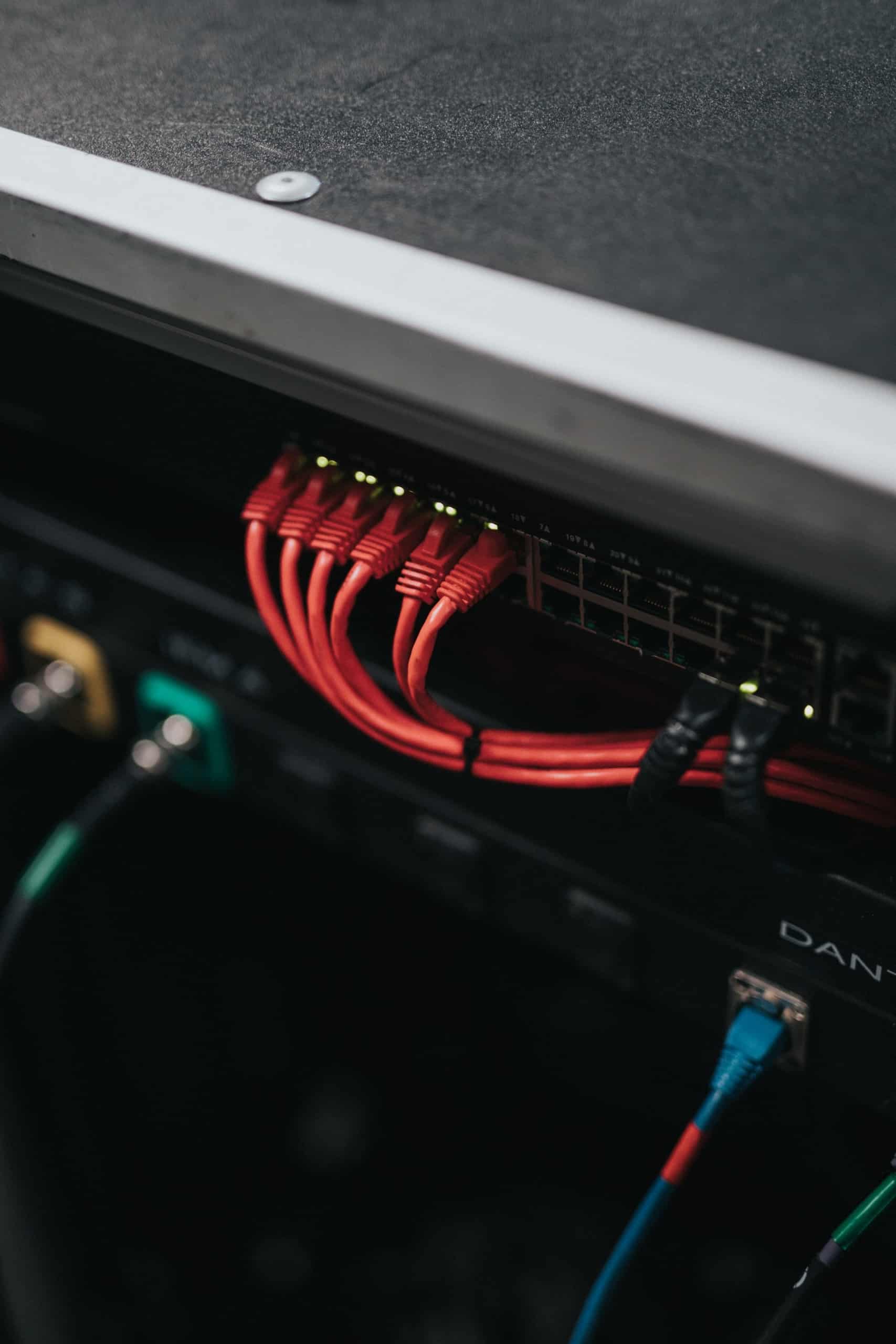
Limitations and Considerations
- Not all bots support typing indicators as a standalone feature.
- Users must grant the bot the necessary permissions to allow it to utilize
sendTyping()
. - Overuse can be spammy and may disrupt chat interactions if not configured properly.
Conclusion
Adding typing indicators to a Discord server can enhance community interaction and make conversations feel more natural. Whether choosing a bot, leveraging API functions, or opting for third-party bots, server administrators have several options to implement this feature efficiently.
Frequently Asked Questions
Can I enable typing indicators directly in Discord server settings?
No, Discord does not provide a built-in option for typing indicators in servers. It is only available in direct messages and group chats.
Will using a typing bot slow down my server?
No, a well-configured bot will not significantly impact server performance. However, excessive API requests may lead to rate limits.
Can I disable typing indicators if I don’t want them?
Yes, most custom bots allow toggling this feature. You can modify the bot settings or remove its permission to manage messages.
Are typing indicators visible to everyone on the server?
Yes, they are visible in channels where the bot is active unless restricted by permission settings.
Which programming languages can be used to create a Discord bot with typing indicators?
Common languages include JavaScript (Discord.js), Python (discord.py), and Java (JDA). Each offers methods to simulate typing indicators.
“`